Summary: in this tutorial, you’ll learn how to use JSON Web Token (JWT) authentication for RESTful API.
What is JWT
Suppose you have a secret code that both you and your friend use to exchange messages. This code allows your friend to trust that the messages are really from you. This is because only you and your friend know the code. In the Web API world, JWT (JSON Web Token) works in a similar fashion.
JWT is like a digital “ID Card” that client and server use to verify identity without needing to store sensitive information such as username/password. JWT allows client and server securely share information between client and server.
Imagine you’re logging into a mobile app. After you enter a valid username and password, the server creates a JWT for you.
The JWT includes your user id and may also contain other information. The server sends JWT back to your mobile app.
Whenever you want to access a protected part of the app, you present this JWT. The server checks the signature to verify its authenticity and reads the information to identify who you are and what you can access
JWT token structure
JWT token has the following structure:
- Header – the header includes the type of token i.e., JWT, and the signing algorithm being used.
- Payload – this is where JWT stores specific information such as user id, expiration time, etc. Think of it like the personal details of your ID card.
- Signature – the signature is created using the header, payload, and a secret key that only the server knows. This ensures that the information in the token is not tampered with.
For example, the following shows a JWT token:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ0b2tlbl90eXBlIjoiYWNjZXNzIiwiZXhwIjoxNjkyNzc4MzQxLCJpYXQiOjE2OTI3NzgwNDEsImp0aSI6IjljODVmMTU4MzIzMzQwOTViYTkxZTNlM2Q2MDA0MWU3IiwidXNlcl9pZCI6MX0.Y1uOK_rxp6_kvDmuULxc82kXgKZPrWsTuLh0Wvg3_Cs
Code language: CSS (css)
If you copy the token to the encoded part of the jwt.io website, you’ll see three parts of its structure:
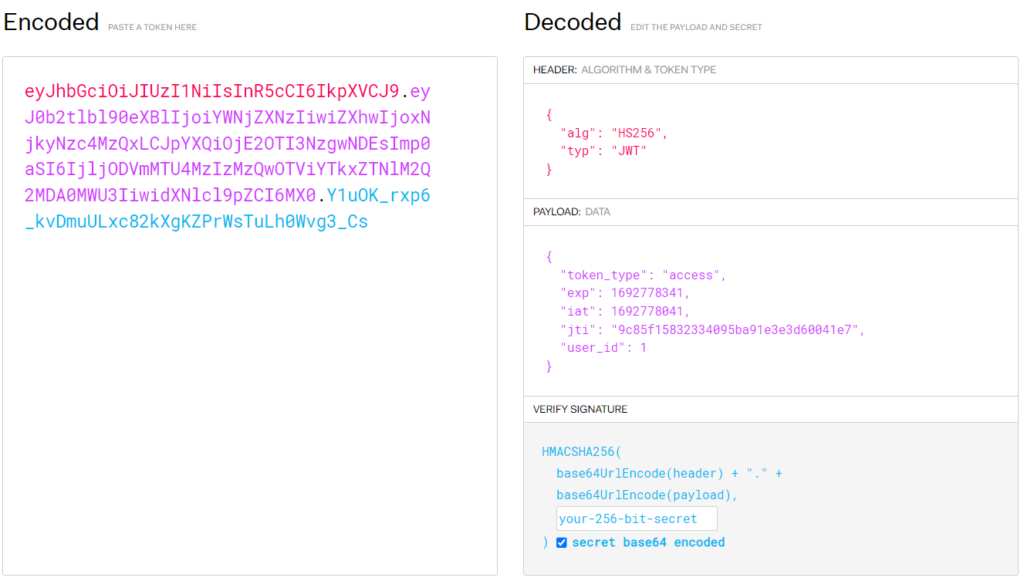
If you modify the JWT token (adding or removing characters), the app will validate the signature and show the result:
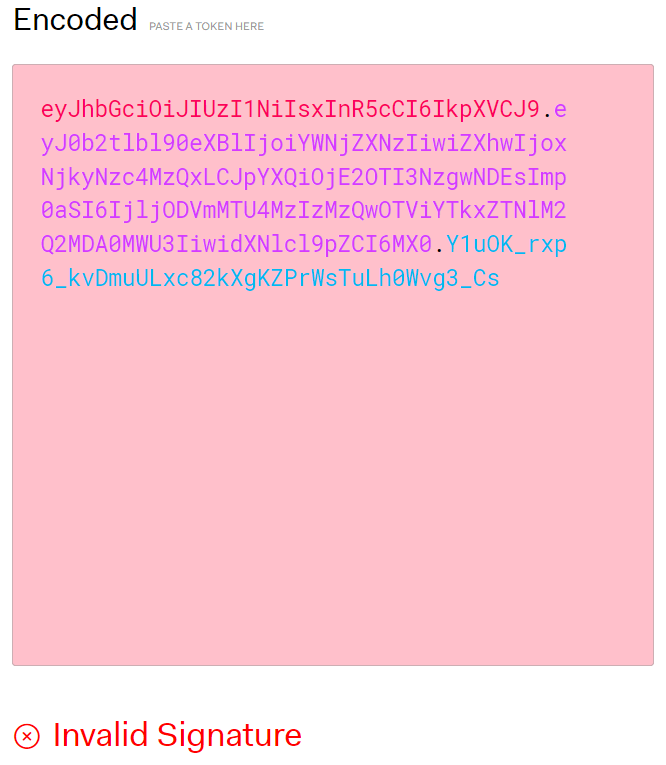
Integrating JWT in the Django REST Framework application
First, install the package djangorestframework-simplejwt
in the current virtual environment:
pip install djangorestframework-simplejwt
Second, add the rest_framework_simplejwt
to the INSTALLED_APPS
of the settings.py
file of the project:
INSTALLED_APPS = [
...,
'rest_framework_simplejwt',
...
]
Code language: Python (python)
Third, configure the project to use the library by adding the rest_framework_simplejwt.authentication.JWTAuthentication
to the list of authentication classes in the settings.py
file:
REST_FRAMEWORK = {
...
'DEFAULT_AUTHENTICATION_CLASSES': (
...
'rest_framework_simplejwt.authentication.JWTAuthentication',
)
...
}
Code language: Python (python)
Fourth, import two views TokenObtainPairView
and TokenRefreshView
from rest_framework_simplejwt.views
into the urls.py
of the project and configure two API endpoints respectively:
from django.contrib import admin
from django.urls import path, include
# jwt
from rest_framework_simplejwt.views import (
TokenObtainPairView,
TokenRefreshView,
)
urlpatterns = [
path('admin/', admin.site.urls),
path('api/v1/', include('api.urls')),
path("auth/", include("rest_framework.urls")),
# jwt
path('api/v1/token/', TokenObtainPairView.as_view(), name='token_obtain_pair'),
path('api/v1/token/refresh/', TokenRefreshView.as_view(), name='token_refresh'),
]
Code language: Python (python)
The endpoint api/v1/token/
allows you to post a valid username and password to obtain a pair of tokens:
- Access token
- Refresh token
Once having the access token, you can add it to the header of an HTTP request to call other APIs. When the access token is expired, you can call the api/v1/token/refresh/
API endpoint to get the new access token.
Getting tokens
To get a pair of tokens, you need to log in using a valid username and password. Open the api/v1/token/
endpoint in the web browser, you’ll see the following page:
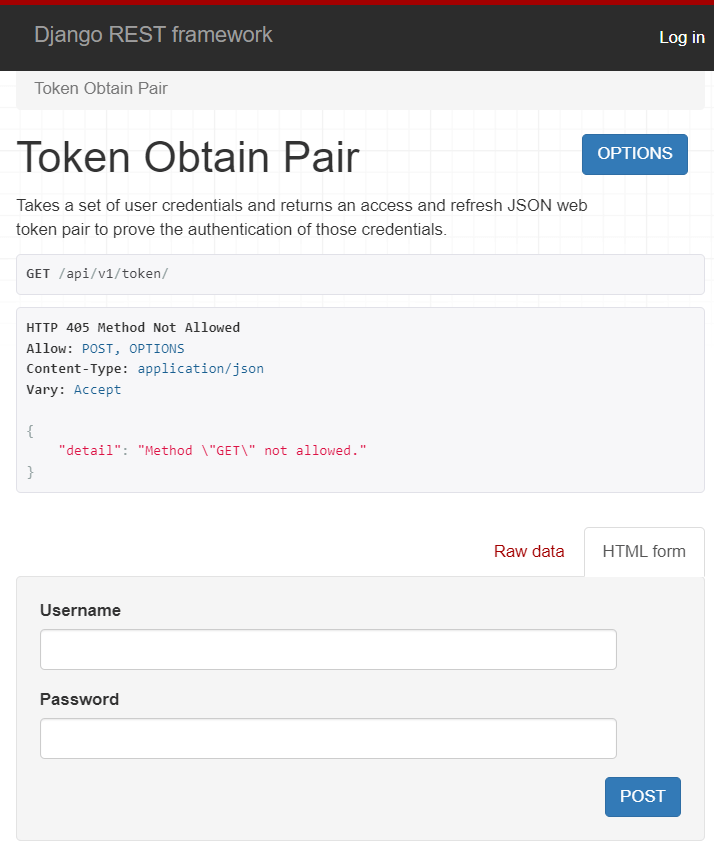
Once you enter a valid username and password and click the POST button:
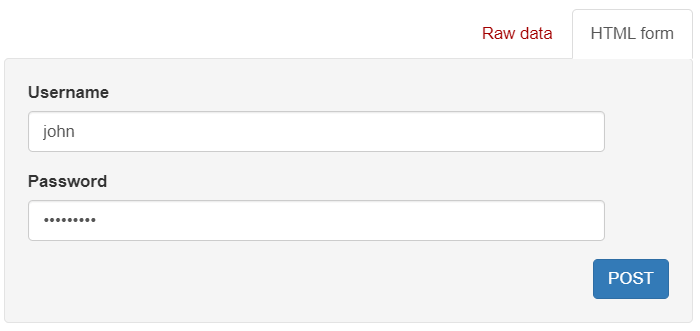
you’ll get the following response:
HTTP 200 OK
Allow: POST, OPTIONS
Content-Type: application/json
Vary: Accept
{
"refresh": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ0b2tlbl90eXBlIjoicmVmcmVzaCIsImV4cCI6MTY5Mjg2NDQ0MSwiaWF0IjoxNjkyNzc4MDQxLCJqdGkiOiJhM2E2MTcyYjZmYjY0YzFlOGJjZGUyMDQ0MjgyNDkwZCIsInVzZXJfaWQiOjF9.8WxI_HcKkm5z6Gx3kCPzpNIkx4nBP52tdiw7-GJv9tA",
"access": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ0b2tlbl90eXBlIjoiYWNjZXNzIiwiZXhwIjoxNjkyNzc4MzQxLCJpYXQiOjE2OTI3NzgwNDEsImp0aSI6IjljODVmMTU4MzIzMzQwOTViYTkxZTNlM2Q2MDA0MWU3IiwidXNlcl9pZCI6MX0.Q_-5AqFxbo1n2sQOOfcdd6ly8XFVT8wJNE-2TQph2ac"
}
Code language: plaintext (plaintext)
Now, you can use the access token to call other APIs without the need of providing the username/password again.
For example, we can use Postman to call the API with the endpoint api/v1/todos/
to get all the todos as follows:
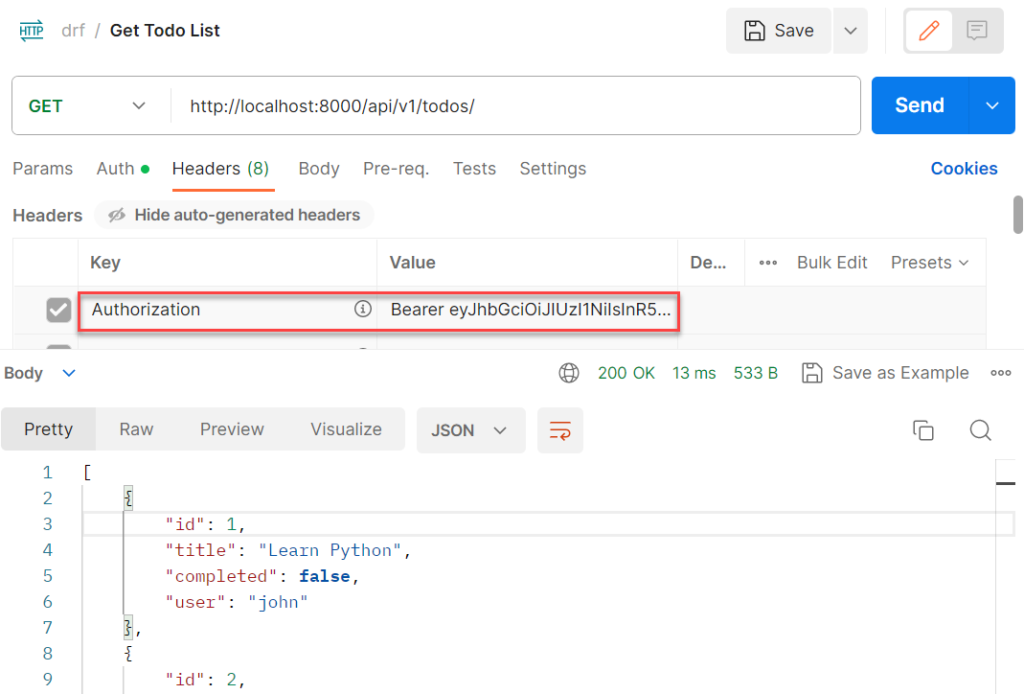
It returns the todos of the user whose username is John
, which is the one that we use to log in to get the tokens.
When the access token is expired, you need to call the API endpoint /api/v1/token/refresh/
with the refresh token to get the new access token.
Open the endpoint /api/v1/token/refresh/
on the web browser, and enter the refresh token, click the POST button:
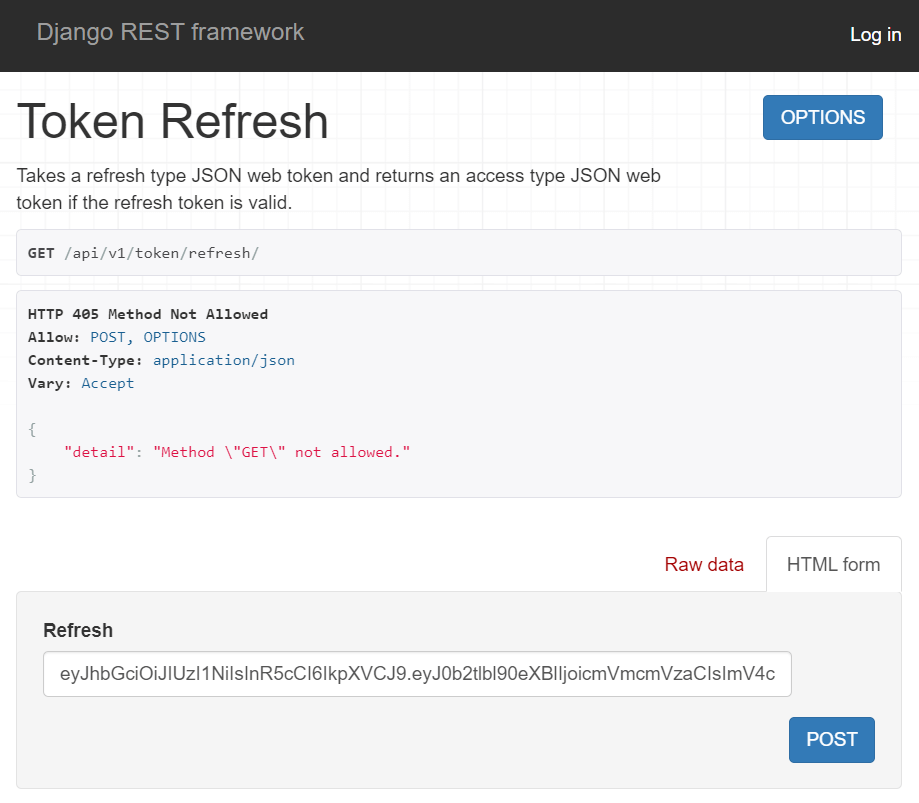
I’ll return you a new access token:
HTTP 200 OK
Allow: POST, OPTIONS
Content-Type: application/json
Vary: Accept
{
"access": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ0b2tlbl90eXBlIjoiYWNjZXNzIiwiZXhwIjoxNjkyNzgzMDk0LCJpYXQiOjE2OTI3NzgwNDEsImp0aSI6IjFiNGRiYjgzZjRhMzRkYTlhMTgzNTY0MzY5M2MxNmQ0IiwidXNlcl9pZCI6MX0.lJeACjeegwoK_SE1NpmXBvKLNEvmahER8Eq-pZ71g7I"
}
Code language: JavaScript (javascript)
And you can use the new access token to access to protected part of the application.
Download the project source code
Click the following link to download the project source code:
Download the project source code.
Summary
- JWT stands for JSON web token.
- JWT provides a secure way that allows server and client securely exchange information.