Summary: in this tutorial, you’ll learn about the Tkinter place
geometry manager to precisely position widgets within its container using the (x, y) coordinate system.
Introduction to Tkinter Place Geometry Manager
The Tkinter place
geometry manager allows you to specify the exact placement of a widget using either absolution or relative positioning.
The placer geometry management gives you fine control over the positioning of widgets by allowing you to:
- Specify coordinates (x, y).
- Use relative positioning based on anchor points.
To use the place
geometry manager, you call the place()
method on the widget like this:
widget.place(**options)
Code language: CSS (css)
1) Absolute positioning
In absolute positioning, you specify the exact x and y coordinates of the widget using the x and y parameters:
widget.place(x=50, y=50)
2) Relative positioning
In relative positioning, you place the widget using the relative coordinates using relx
and rely
parameters:
For example, the following place the widget in the center of its parent:
widget.place(relx=0.5, rely=0.5, anchor=CENTER)
3) width and height
The place geometry manager allows you to set the width and height of the widget via the width
and height
paramaters:
widget.place(width=120, height=60)
Alternatively, you can use relative sizing concerning the parent container. For example, the following code sets the widget’s width and height to 50% of the parent’s dimensions:
widget.place(relwidth=0.5, relheight=0.5)
The relwidth
and relheight
has a value of a floating-point number between 0.0 and 1.0. This value represents a fraction of the width and height of the parent container.
4) Anchor
The anchor
parameter determines which part of the widget is positioned at the given coordinates.
The anchor
parameter accepts values such as:
'n'
,'ne'
,'e'
,'se'
,'sw'
,'w'
,'nw'
: These constants represent the cardinal and intercardinal directions (north, northeast, east, southeast, south, southwest, west, northwest).'center'
: This value instructs theplace()
method to position the center of the widget at the specified coordinates.
The default value of the anchor is 'nw'
which instructs the place()
method to position the top left of the widget at the specified coordinates.
For example, the following code places the widget in the center of the container widget:
widget.place(relx=0.5, rely=0.5, anchor='center')
Code language: JavaScript (javascript)
Tkinter place geometry manager examples
Let’s take some examples of using the Tkinter place geometry manager.
1) Absolute positioning example
The following example uses the place geometry manager to place a label at (0,0) with a width of 60 and height of 120:
import tkinter as tk
root = tk.Tk()
root.title('Tkinter Place Geometry Manager')
root.geometry("600x400")
label1 = tk.Label(master=root, text="Place",bg='red',fg='white')
label1.place(x=0,y=0,width=120, height=60)
root.mainloop()
Code language: JavaScript (javascript)
Output:
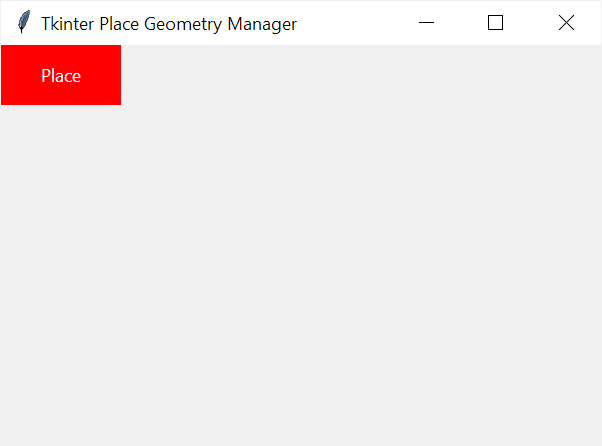
2) Relative positioning example
The following program places a Label widget with its top-left corner at the center of the window:
import tkinter as tk
root = tk.Tk()
root.title('Tkinter Place Geometry Manager')
root.geometry("600x400")
label1 = tk.Label(master=root, text="Place",bg='red',fg='white')
label1.place(relx=0.5, rely=0.5, width=100, height=50)
root.mainloop()
Code language: JavaScript (javascript)
Output:
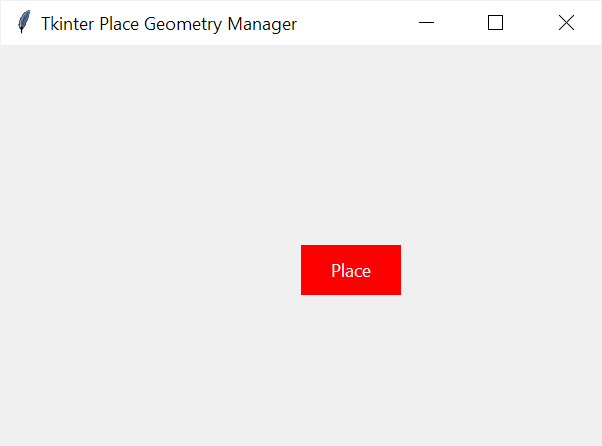
3) Using the anchor point
The following example places the center point of the Label widget at the center of the window:
import tkinter as tk
root = tk.Tk()
root.title('Tkinter Place Geometry Manager')
root.geometry("600x400")
label1 = tk.Label(master=root, text="Place",bg='red',fg='white')
label1.place(relx=0.5, rely=0.5, width=100, height=50, anchor=tk.CENTER)
root.mainloop()
Code language: JavaScript (javascript)
Output:
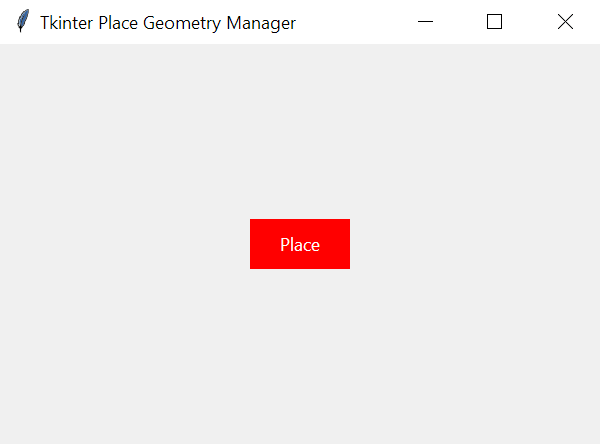
Summary
- Use the Tkinter
place
geometry manager to precisely position widgets within its container using the (x, y) coordinate system.