Summary: in this tutorial, you’ll learn about how Django sessions work and how to set various settings for the session.
Introduction to Django sessions #
Django has a session framework that supports both anonymous and user sessions. Django uses the session middleware to send and receive cookies.
The following picture illustrates how the Django sessions work:
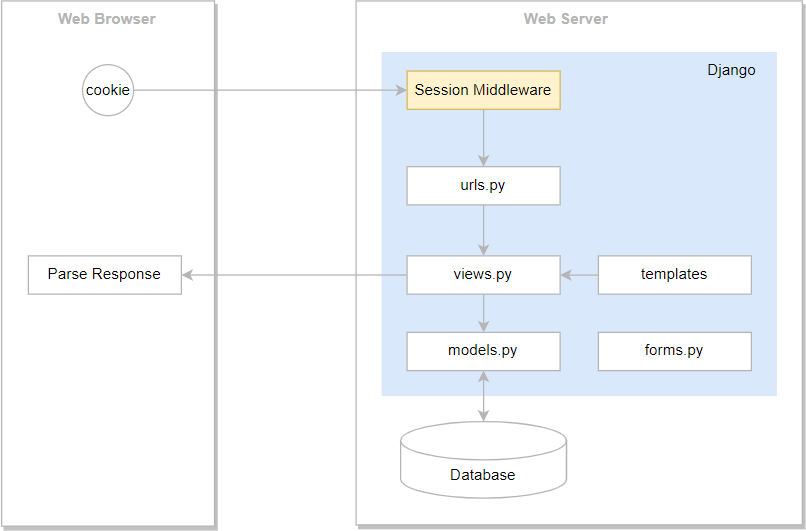
When a web browser makes the first HTTP request to the web server, the session middleware starts a new session. The session middleware generates a large and random number which is called a session identifier and sends it back to the web browser as a cookie.
For the subsequent requests, the session middleware matches the value sessionid
in the cookie sent by the web browser with the session identifier stored on the web server and associates the session data with the HTTP request object.
To use sessions, you need to ensure that the MIDDLEWARE
settings of your project (settings.py
) contain the session middleware like this:
MIDDLEWARE = [
# other middleware
'django.contrib.sessions.middleware.SessionMiddleware',
# ...
]
Code language: Python (python)
The session middleware is added to the MIDDLEWARE
by default when you create a new project using the startproject
command.
The session middleware enables sessions via the session
property of the request object (HttpRequest
):
request.session
Code language: Python (python)
The request.session
is a dictionary that allows you to store and retrieve session data. The request.session
accepts any object that can be serialized to JSON by default.
Unlike other objects, the request.session
persists from one HTTP request to the next request.
To set a variable in the session, you can use the request.session
like this:
request.session['visit'] = 1
Code language: Python (python)
This statement sets the visit variable to 1.
To retrieve a session key, you use the get()
method of the request.session
object:
request.session.get('visit')
Code language: Python (python)
To delete a key in the session, you use the del
statement:
del request.session['visit']
Code language: Python (python)
Setting Django sessions #
By default, Django stores session data in a database using the Session
model of the django.contrib.sessions
application. However, you can choose other session engines using the SESSION_ENGINE
.
Django provides you with the following options for storing session data:
Options | Description |
---|---|
Database sessions | Store session data in the django_session of the database. This is the default engine. |
File-based sessions | Store session data in the filesystem. |
Cached sessions | Store session data in a cache backend. To set the cache backend, you use the CACHES setting. |
Cached database sessions | Store session data in a write-through cache and database. If the data is not in the cache, Django will read the session data from the database. |
Cookie-based sessions | Store session data in the cookies that are sent to the web browser. |
Note that a cache-based session engine provides better performance in comparison with other session engines.
Django supports Memcached out of the box. In addition, you can find a third-party package for managing cache backends for Redis and other cache systems.
Besides the SESSION_ENGINE
, Django allows you to customize sessions with specific settings. The following table list the most important ones:
Session settings | Description |
---|---|
SESSION_COOKIE_AGE | The duration of session cookies in session. The default is two weeks (1,209,600 seconds) |
SESSION_COOKIE_DOMAIN | Set the domain for session cookies. |
SESSION_COOKIE_HTTPONLY | Set to True to prevent JavaScript from accessing the session cookie. It defaults to True, which increases security against user session hijacking. |
SESSION_COOKIE_SECURE | Set to True to indicate that the cookie should only be sent if the connection is an HTTPS connection. It defaults to False. |
SESSION_EXPIRE_AT_BROWSER_CLOSE | Set to True to expire the session when you close the browser. Its default value is False. If you set this to True, the SESSION_COOKIE_AGE won’t have any effect. |
SESSION_SAVE_EVERY_REQUEST | Set to True to save the session and update session expiration to the database on every request. It defaults to False. |
Django sessions example #
First, add a URL to the urlpatterns
in the urls.py
file:
urlpatterns = [
path('visit/', views.count_visit, name='visit')
]
Code language: Python (python)
When you navigate to http://localhost:8000/visit
, the count_visit
function in views.py will execute.
Second, define the count_visit()
function in the views.py
file:
def count_visit(request):
visit = request.session.get('visit',0) + 1
request.session['visit'] = visit
return HttpResponse(f"Visit count:{request.session['visit']}")
Code language: Python (python)
The count_visit()
function uses the request.session
to increase the visit
variable each time you visit the http://localhost:8000/visit
URL. It also displays the current value of the visit
variable on the web page:
If you view the cookies in the web browser, you’ll see a cookie with the name sessionid:
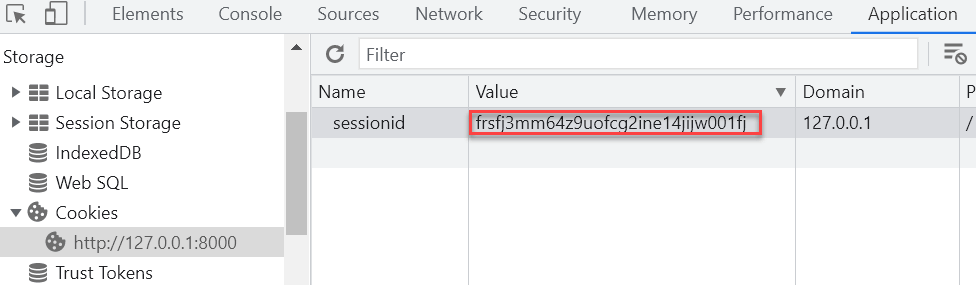
The value of the sessionid is corresponding with the session_key in the django_session table:

The session data is a string encoded using base64. To analyze it, you use the following code:
from base64 import b64decode
data = base64decode('eyJ2aXNpdCI6MTF9:1ov84c:qngX5Woil1EDwGGylot0OrQtche6734UOApKJ4yp-BA')
print(data)
Code language: Python (python)
Output:
b'{"visit":11}\xd6\x8b\xfc\xe1\xca\xa7\x81~V\xa2)u\x10<\x06\x1b)h\xb7C\xabB\xd7!{\xae\xf7\xe1C\x80\xa4\xa2x\xca\x90@'
Code language: plaintext (plaintext)
Summary #
- A session is a variable that lives across requests.
- Django uses session middleware to manage sessions.
- Use
request.session
to manage session data.