Summary: in this tutorial, you’ll learn how to use the Django isnull
to check whether a value is NULL
or not.
Introduction to Django isnull #
We’ll use the Employee
and Contact
models from the HR
application for the demonstration. The Emloyee
and Contact
models map to the hr_employee
and hr_contact
tables:
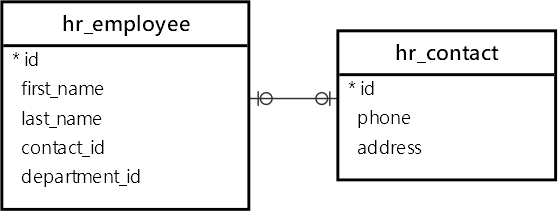
In relational databases,
denotes missing information. For example, if you don’t know the contact of an employee, you can use NULL
for the contact of the employee at the time of recording.NULL
NULL
is special because you cannot use the =
operator to compare a value with it. Instead, you use the IS
operator.
For example, to check if the value in the contact_id
is NULL
or not, you use the IS
operator as follows:
contact_id IS NULL
Code language: Python (python)
The IS
returns true if the NULL
contact_id
is
or false otherwise.NULL
To negate the IS
operator, you can use the NOT
operator like this:
contact_id IS NOT NULL
Code language: Python (python)
Django uses isnull to check if a value is NUL
or not:
Entity.objects.filter(field_name__isnull=True)
Code language: Python (python)
The filter()
method returns all instances with the field_name
is NULL
. To negate the isnull
, you compare it with False
instead:
Entity.objects.filter(field_name__isnull=False)
Code language: Python (python)
In this case, the filter()
returns all instances of the Entity whose field_name
is not NULL
.
Django isnull example #
The following example uses the isnull
to get employees who do not have contacts:
>>> Employee.objects.filter(contact_id__isnull=True)
SELECT "hr_employee"."id",
"hr_employee"."first_name",
"hr_employee"."last_name",
"hr_employee"."contact_id",
"hr_employee"."department_id"
FROM "hr_employee"
WHERE "hr_employee"."contact_id" IS NULL
Code language: Python (python)
The generated query use IS NULL
operator to compare the contact_id
with NULL
.
The following example uses the isnull
to get all employees who have contacts:
>>> Employee.objects.filter(contact_id__isnull=False)
SELECT "hr_employee"."id",
"hr_employee"."first_name",
"hr_employee"."last_name",
"hr_employee"."contact_id",
"hr_employee"."department_id"
FROM "hr_employee"
WHERE "hr_employee"."contact_id" IS NOT NULL
Code language: Python (python)
In this case, the generated query uses the IS NOT NULL
to compare the values in the contact_id
column with NULL
.
Summary #
- Use Django
isnull
to check if a value isNULL
or not.