Summary: in this tutorial, you’ll learn how to use the Django QuerySet
exists()
method to check if a
contains any rows.QuerySet
Introduction to the Django QuerySet exists() method #
Sometimes, you want to check if a query contains any rows. To do it, you use the exists()
method of the QuerySet
object.
The exists()
method returns True
if the QuerySet
contains any rows or False
otherwise.
Behind the scenes, the exists()
will attempt to perform the query in the fastest way possible. However, the query will be nearly identical to a regular QuerySet
query.
Suppose you have a QuerySet
and want to check if it has any objects. Instead of doing this:
if query_set:
print('the queryset has at least one object')
Code language: Python (python)
…you should use the exists()
because it is a little bit faster:
if query_set.exists():
print('the queryset has at least one object')
Code language: Python (python)
Django 4.1 added the a
which is an asynchronous version of exists()
exists()
.
Django exists() method example #
We’ll use the Employee
model for the demonstration. The Employee
model maps to the hr_employee
table in the database:
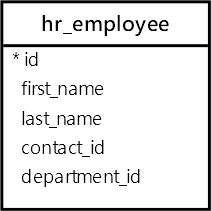
First, run the shell_plus
command:
python manage.py shell_plus
Code language: plaintext (plaintext)
Second, find the employees whose first names start with the letter J
:
>>> Employee.objects.filter(first_name__startswith='J').exists()
SELECT 1 AS "a"
FROM "hr_employee"
WHERE "hr_employee"."first_name"::text LIKE 'J%'
LIMIT 1
Execution time: 0.000000s [Database: default]
True
Code language: SQL (Structured Query Language) (sql)
Note that Django generated the SQL based on the PostgreSQL. If you use other databases, you may see a slightly different SQL statement.
In this example, the exists()
method returns True. It selects only the first row to determine whether the QuerySet
contains any row.
If you do not use the exists()
method, the QuerySet
will get all the rows from the hr_employee
table:
>>> qs = Employee.objects.filter(first_name__startswith='J')
>>> print(qs.query)
SELECT "hr_employee"."id",
"hr_employee"."first_name",
"hr_employee"."last_name",
"hr_employee"."contact_id",
"hr_employee"."department_id"
FROM "hr_employee"
WHERE "hr_employee"."first_name"::text LIKE J%
ORDER BY "hr_employee"."first_name" ASC,
"hr_employee"."last_name" ASC
Code language: SQL (Structured Query Language) (sql)
Summary #
- Use the Django
exists()
method to check if aQuerySet
contains any rows.