Summary: in this tutorial, you will learn about the enumerate()
function and how to use it to enumerate a sequence, iterator, or any other object that supports iteration.
Introduction to the Python enumerate() function
The following example defines a list of fruits and uses the for-in loop to iterate over the list elements and print them out:
fruits = ['Apple', 'Orange', 'Strawberry']
for fruit in fruits:
print(fruit)
Code language: Python (python)
Output:
Apple
Orange
Strawberry
Code language: Python (python)
It works fine as expected.
Sometimes, you want to add indices to the output. To do that, you may come up with a loop counter like this:
fruits = ['Apple', 'Orange', 'Strawberry']
index = 1
for fruit in fruits:
print(f'{index}.{fruit}')
index += 1
Code language: Python (python)
Output:
1.Apple
2.Orange
3.Strawberry
Code language: Python (python)
In this example, the index keeps track of the indices. This example also works as expected. But Python has a better solution with the enumerate()
function:
fruits = ['Apple', 'Orange', 'Strawberry']
for index, fruit in enumerate(fruits, start=1):
print(f'{index}.{fruit}')
Code language: Python (python)
Output:
1.Apple
2.Orange
3.Strawberry
Code language: Python (python)
With the enumerate()
function, you can achieve the same result with less code. Also, you don’t need to keep track of the index by increasing it in each iteration.
The enumerate()
is a built-in function with the following syntax:
enumerate(iterable, start=0)
Code language: Python (python)
The enumerate()
function accepts two arguments:
iterable
is a sequence, an iterator, or any object that supports the iterable protocol.start
is the starting number from which the function starts counting. It defaults to zero.
The enumerate()
function returns an enumerate object. The __next__()
method of the returned enumerate object returns a tuple that contains a count and the value obtained from iterating over the iterable.
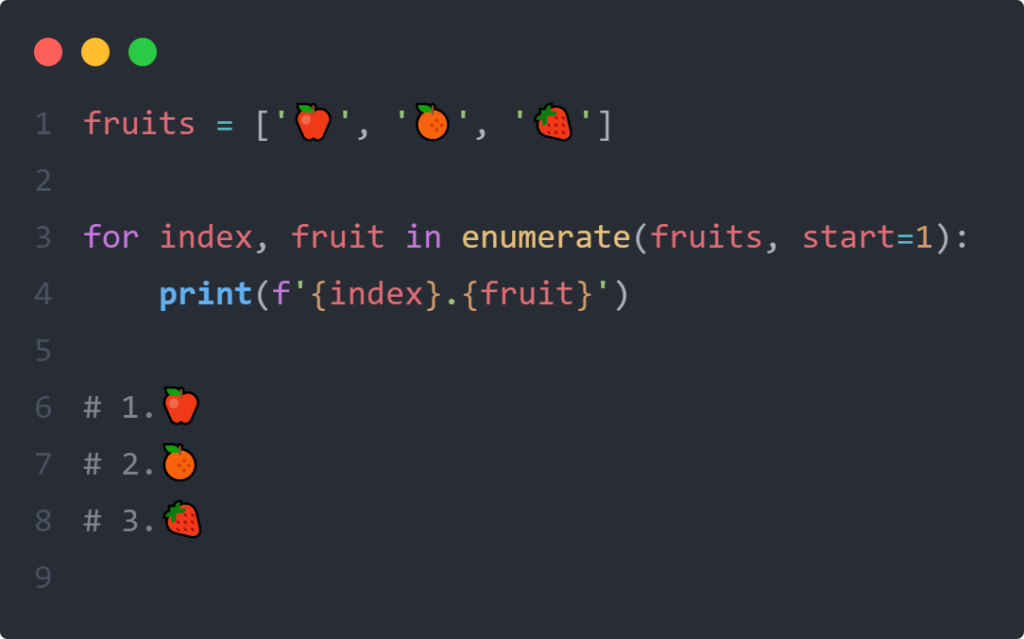
See the following example:
fruits = ['Apple', 'Orange', 'Strawberry']
e = enumerate(fruits, start=1)
print(type(e))
Code language: Python (python)
In this example, the enumerate()
function returns e
which is an instance of the enumerate
class:
<class 'enumerate'>
Code language: Python (python)
The enumerate class has the __next__()
method that returns a tuple:
fruits = ['Apple', 'Orange', 'Strawberry']
e = enumerate(fruits, start=1)
print(e.__next__())
Code language: Python (python)
Output:
(1, 'Apple')
Code language: Python (python)
If you keep calling the __next__()
method, it’ll return the next counting number with the value from the list:
fruits = ['Apple', 'Orange', 'Strawberry']
e = enumerate(fruits, start=1)
print(e.__next__())
print(e.__next__())
print(e.__next__())
Code language: Python (python)
Output:
(1, 'Apple')
(2, 'Orange')
(3, 'Strawberry')
Code language: Python (python)
When all items in the list are iterated, calling the __next__()
method will raise a StopIteration
exception:
fruits = ['Apple', 'Orange', 'Strawberry']
e = enumerate(fruits, start=1)
print(e.__next__())
print(e.__next__())
print(e.__next__())
print(e.__next__()) # StopIteration
Code language: Python (python)
The enumerate()
is functionally equivalent to the following function:
def enumerate(iterable, start=0):
index = start
for item in iterable:
yield index, item
index += 1
Code language: Python (python)
Summary
- Use Python
enumerate()
function to get items of an iterable with a counter.